My Project: Travel time bot with Google Maps API and Python - Part 2 - Making the travel data useful
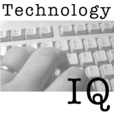
Summary: In Part 1 of this series, I showed you how I found the travel time data I wanted and how I figured out how JSON data files function and how to pull out just the data I need. Now that I have this data, I needed to learn a bit of Python coding to extract is and use it in calculations and present it to the screen and other in other ways. Learning Python As has been the case throughout my technology work history, I learn on-demand. For me, this means coming up against a problem and then researching how to solve that problem on the Internet, adapting sample code and finally getting it all to work. That was exactly what happened in the project. I was not very familiar with Python, so each time I needed to accomplish a task I would head over to Google Search (which almost always led to me Stack Overflow) to find the answer. Let me walk you through my Python code and explain how it works and a little about how I developed it.Learn more about Python with these books from Amazon.comMore Python Books Python Modules Let’s begin at the top. First, I needed to figure out what Python modules I would need to accomplish all I wanted with the Travel Time program. #!/usr/bin/env python import urllib import simplejson from os import system import os import time The urllib module gave me the tools to access web URLs within the program and request web pages — or in this case — a JSON file served up from the Google Maps API server. simplejson — as you might imagine — provided the code to easily manipulate JSON by converting it into easily accessible arrays of information. os and system modules allow me to call on basic operating system utilities like curl and — since I am running on a Mac — say, which speaks the information out loud. The time module provides me the ability to add wait times and get the current data and time. Looking back at the code now, I realize I don’t really use it in this version. I originally had the script set to run every 5 minutes or so, instead of on-demand, so that functionality is not longer needed. Getting the JSON Data Next, it was time to get the actual current JSON data from the Google Maps API. Each time this is called, it returns the current travel time based on distance and also traffic loading. Note: I have removed my own personal API key from the URL. You’ll need to add that in in — along with your start and end coordinates — to make this useful to you. # Google Maps API URL api_url = 'https://maps.googleapis.com/maps/api/directions/json?origin=34.178026,-118.4528837&destination=34.057835,-117.8217123&departure_time=now&key=<INSERTKEYHERE> # Get JSON data from Google Maps API response = urllib.urlopen(api_url) data = simplejson.load(response) The api-url variable is simply an easily modifiable placeholder for the url you might use. This is then used to in the call to urllib to pull the actual data from that API url. Note that I have no error checking in whether this actually succeeded or not. This is something you would probably want to add. The data returned by the urllib call is stuffed into a variable called response. This response variable is then used in the call to simplejson which creates an array called data which has all the various key-value pairs sorted out and made easily accessible. My initial version of the program used simple text commands to hack away at the data I needed and was much more difficult and brute force. Once I learned how simplejson worked, though. it open the doors to not only accessing API data for this program, but any API data in JSON format from any API. This was a big step in my learning, for sure. Finding the data I needed in the simplejson array # Get current duration of trip duration = (data['routes'][0]['legs'][0]['duration_in_traffic']['value']) # Get current summary name for trip desc = (data['routes'][0]['summary']) You may remember from Part 1 how I used the JSONViewer to give me a clearer, hierarchical understanding of the returned JSON file. These